1
/
of
1
เว็บปั่นสล็อตฟรี
เว็บปั่นสล็อตฟรี พีจี ทดลองเล่น แตกง่าย ไม่มีขั้นต่ำ เล่นง่ายจ่ายจริง
เว็บปั่นสล็อตฟรี พีจี ทดลองเล่น แตกง่าย ไม่มีขั้นต่ำ เล่นง่ายจ่ายจริง
Regular
price
900 ฿ THB
Regular
price
Sale
price
900 ฿ THB
Unit price
/
per
ทดลองเล่นเกมสล็อตฟรี DuangDeeBoss เว็บสล็อตเว็บตรงที่ครบเครื่องที่สุดในปี 2024 สล็อตเว็บตรง ที่มีอัตราการชนะสูงมากที่สุดเป็นอันดับ 1 แตกง่ายทุกเกม และ สล็อตทดลอง - สล็อตเว็บตรง.
ทดลองเล่นเกมสล็อตฟรี DuangDeeBoss เว็บสล็อตเว็บตรงที่ครบเครื่องที่สุดในปี 2024 สล็อตเว็บตรง ที่มีอัตราการชนะสูงมากที่สุดเป็นอันดับ 1 แตกง่ายทุกเกม และ สล็อตทดลอง - สล็อตเว็บตรง. นอกจากนี้ยังมีการให้บริการด้วยระบบที่เสถียรและเข้าใจง่าย ทำให้ผู้ใช้ไม่เพียงแต่ได้รับความสนุกสนานที่ล้ำหน้า แต่ยังรู้สึกความปลอดภัยในขณะเล่น ด้วยการบริการลูกค้าที่พร้อมตอบทุกข้อซักถาม 24 ชั่วโมง ทำให้ DuangDeeBoss กลายเป็นที่นิยมอย่างยิ่งในหมู่ผู้เล่นเกมสล็อตในปี 2024.
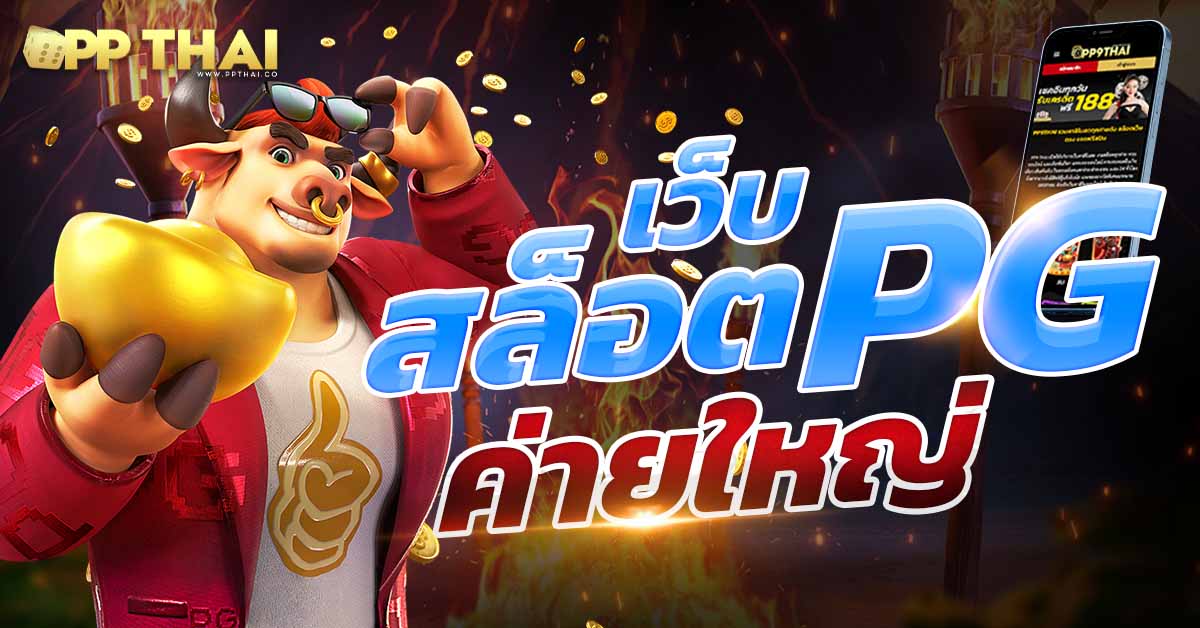